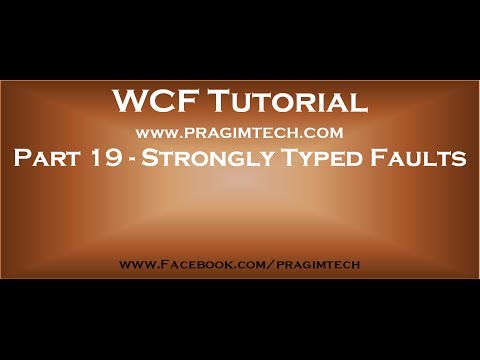
http://www.youtube.com/user/kudvenkat...
Part 19 - Creating and throwing strongly typed SOAP faults
This is continuation to Part 18, please watch Part 18 before proceeding.
In Part 18, we discussed throwing a generic SOAP fault using FaultException class. Instead of throwing a generic SOAP fault, we can create strongly typed SOAP faults and throw them. Creating our own strongly typed SOAP faults allow us to include any additional custom information about the exception that has occurred. Here are the steps to create a strongly typed SOAP fault.
Step 1: Riglt click on the CalcualtorService and add a class file with name = DivideByZeroFault.cs. Copy and paste the following code. DivideByZeroFault is a strongly typed fault.
using System.Runtime.Serialization;
namespace CalculatorService
{ [DataContract] public class DivideByZeroFault { [DataMember] public string Error { get; set; } [DataMember] public string Details { get; set; } }
}
Step 2: Make the following changes to ICalculatorService.cs. Notice the change highlighted in yellow. We have used FaultContract attribute to specify that the Divide() method can throw DivideByZeroFault.
using System.ServiceModel;
namespace CalculatorService
{ [ServiceContract] public interface ICalculatorService { [FaultContract(typeof(DivideByZeroFault))] [OperationContract] int Divide(int Numerator, int Denominator); }
}
Step 3: Copy and paste the following code in CalculatorService.cs. Notice that instead of throwing a generic SOAP fault, we are throwing a strongly typed SOAP fault using FaultException[T] class, where [T] is DivideByZeroFault.
using System.ServiceModel;
using System;
namespace CalculatorService
{ public class CalculatorService : ICalculatorService { public int Divide(int Numerator, int Denominator) { try { return Numerator / Denominator; } catch (DivideByZeroException ex) { DivideByZeroFault divideByZeroFault = new DivideByZeroFault(); divideByZeroFault.Error = ex.Message; divideByZeroFault.Details = "Denominator cannot be ZERO"; throw new FaultException[DivideByZeroFault](divideByZeroFault); } } }
}
As we have changed the WCF service, update the service reference in the client application.
Step 4: Finally in the client application, modify the catch block as shown below.
catch (FaultException[CalculatorService.DivideByZeroFault] faultException)
{ label1.Text = faultException.Detail.Error + " - " + faultException.Detail.Details;
}
So in short, to create a strongly typed SOAP fault
1. Create a class that represents your SOAP fault. Decorate the class with DataContract attribute and the properties with DataMember attribute.
2. In the service data contract, use FaultContractAttribute to specify which operations can throw which SOAP faults.
3. In the service implementation create an instance of the strongly typed SOAP fault and throw it using FaultException[T].
soapbox Part 19 Creating and throwing strongly typed SOAP faults | |
110 Likes | 110 Dislikes |
50,335 views views | 524K followers |
Education | Upload TimePublished on 27 Jan 2014 |
Related keywords
asp.net core docker,strongly infatuated crossword clue,wcf vs web api,soap and glory,ado.net core,sql server management studio,webkinz,webadvisor,craigslist nj,wcf one piece,asp.net core 3,ajax players,weber grill parts,webtoon,tutorials by hugo,csharp corner,mvc design pattern,asp.net machine account,servers for minecraft,asp.net core dependency injection,ado.net tutorial,services angular,ajax ontario,tutorial definition,tutorial on excel,asp.net mvc tutorial,soapstone countertops,asp.net cos'è,csharp assembly,tutorialspoint python,sql join,services briefcase,asp.net core web api,ajax jquery,wccftech,craigslist ny,asp.net zero,csharp foreach,server memes,soap nuts,sql date format,services online,chase,serverless architecture,server resume,wcf c#,server books,tutorialspoint javascript,mvcc connect,ado.net mysql,services technologies gps,ajax deadpool,server jobs,soap opera,cvs,website,mvc tutorial,costco hours,wcf service application,tutorialspoint spring,serverless,wcf soap,wcf cat,cool math games,wcf test client,strongly connected graph,services & training hse colombia sas,servicestack,citibank,asp.net core identity,sql union,soap opera digest,ajax parking,sql database,asp.net core logging,mvconnect,cunyfirst,asp.net guida,wcf nba,csharp download,wcfi foundation,csharp online,wcf authentication,tutorials near me,http://asp.net,server 2019,chernobyl,ado.net descargar,web of dreams,serverminer,ajax cleaner,ado.net visual studio 2019,tutorial for windows 10,webassign,soap note example,ado.net vs entity framework,strongly typed python,ado.net visual studio 2017,csharp list,strongly agree,sql like,asp.net mvc,soap dish,asp.net core tutorial,sqlite,wcfm,ajax roster,strongly built,tutorial gateway,mvc architecture,strongly powerfully,http://ado.net,asp.net core mvc,ajax soccer,server hosting,strongly advise,wcfi,strongly agree scale,ajax dish soap,soap molds,capital one,server rack,tutorialspoint html,soap shoes,csharp interface,craigslist,strongly recommend,webroot,tutorialspoint reactjs,ajax request,wcf dragon ball,asp.net core 2.2,soap note,strongly worded letter,tutorialspoint python 3,sql developer,webster,services transmission company sas,sql group by,asp.net core signalr,services manager,mvc framework,ajax paving,mvc near me,tutorialspoint spring boot,strongly disagree,mvc map,csharp online compiler,asp.net download,sql between,ado.net c# pdf,services tag dell,wcf 2019 nba,csharp switch,ado.net ventajas y desventajas,csharpstar,wcf tutorial,soap dispenser,tutorialspoint,strongly meaning,ajax meaning,csharp-video-tutorials.blogspot,tutorials dojo,tutorial mission gta online,central park 5,csharp string format,ado.net c#,asp.net core github,server status,ajax fc,server jobs nyc,asp.net core swagger,sql formatter,credit karma,services group,server error in '/' application,services windows,asp.net core 3.0,sql injection,tutorialspoint c#,wcf ria services,calculator,ado.net entity data model,sql insert,tutorialspoint tableau,soapcentral,services google play apk,sqlyog,asp.net core 3 release date,sql server,server job description,strongly advises crossword clue,tutorials by a,servicenow,webcam,soap making supplies,mvc hours,webmd symptom,csharp array,soap she knows,csharp enum,ajax call,asp.net core 2. guida completa per lo sviluppatore,asp.net core,server pro,server status ffxiv,strongly rebuke,cheap flights,webcrims,asp.net core hosting,services sas,tutorialspoint java,soap dirt,tutorialspoint java compiler,strongly connected components,webmd,csharp to json,college football,ado.net dataset,csharp dictionary,tutorial meaning,cnn,website builder,tutorial.mc-complex,tutorialspoint sql,asp.net web api,server side rendering,weber grills,sql server 2017,tutorialspoint spark,mvc nj,ado.net oracle,asp.net core download,csharp to vb.net,webster bank,webstaurant,tutorial systems,tutorialsystems,tutorial teacher,ajax post,services fms publish announcement,services.msc no abre,ajax jersey,csharp operator,asp.net core razor pages,server duties,asp.net core environment variables,soap brows,tutorial on variational autoencoders,csharp random,century 21,services consultores,services consulting,mvcsd,services.msc,mvcsc,ado.net pdf,asp.net core configuration,ajax marvel,tutorial synonym,sql update,asp.net tutorial,mvc medical,ado.net entity data model visual studio 2019,wcfs international curriculum,mvc2,ado.net entity data model visual studio 2017,chase online,wcf api,costco,server jobs near me,strongly scented herb,webex,sql meaning,strongly synonym,sql tutorial,sql commands,strongly believe synonym,ado.net entity framework,strongly typed,ajax tavern,tutorialsteacher,ajax javascript,services desk,soapui,ajax greek,strongly against,csharp tutorial,mvc pattern,ado.net sql server,ado.net connection,asp.net identity,mvcu,asp.net core middleware,wcf web service,soap2day,mvc webadvisor,tutorial music,web store,mvcc,webmail,mvci,soapstone,mvctc,
Không có nhận xét nào:
Đăng nhận xét